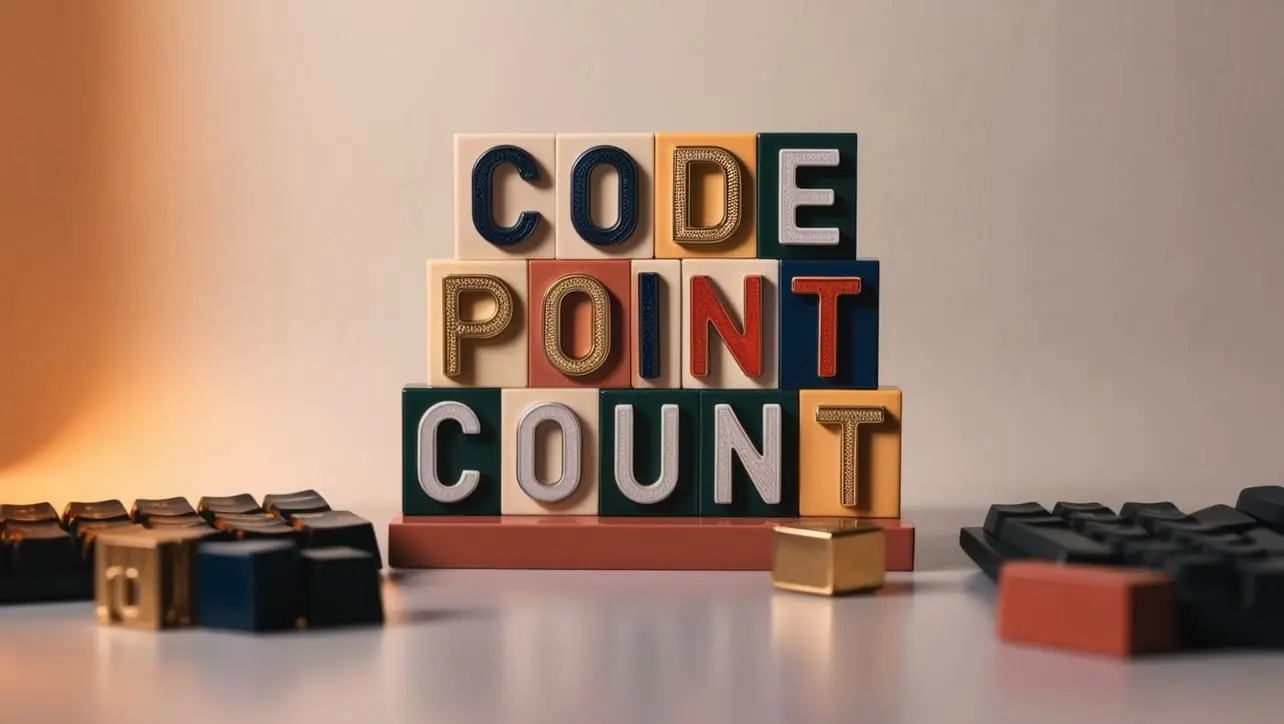
Java Basic
Java Introduction
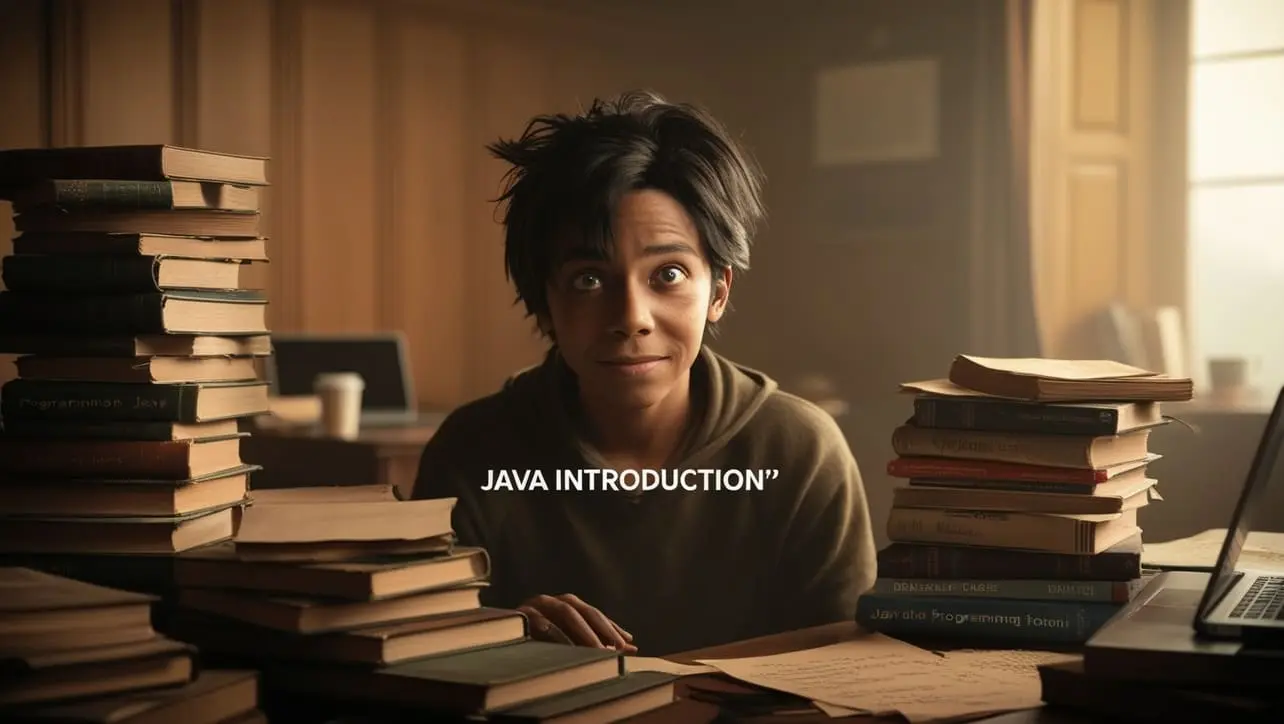
Photo Credit to CodeToFun
What is Java? #
Java is a high-level, object-oriented programming language that is platform-independent, meaning that Java code can run on any system that has a Java Virtual Machine (JVM) installed.
It is commonly used to build enterprise-level applications, mobile applications, and web applications, and it has a large ecosystem of libraries and frameworks to help developers build complex applications more efficiently.
Who is the Father of Java?
James Gosling is considered the "Father of Java" for his significant contributions to the design and development of the programming language while he was working at Sun Microsystems in the mid-1990s.
How Java Works?
Java works by compiling code written in the Java programming language into bytecode, which can then be executed on any system that has a Java Virtual Machine (JVM) installed.
The JVM acts as a virtual machine, running the bytecode on the target system and translating it into machine language instructions that can be executed by the computer's processor. This allows Java code to be platform-independent, meaning that it can run on any system that has a JVM installed, regardless of the underlying hardware and operating system.
Java is also notable for its automatic memory management, which helps to simplify memory management for developers and reduce the risk of memory-related errors.
Java has a wide range of libraries and frameworks that provide developers with tools and resources to help them build complex applications more efficiently.
Is Java Easy to Learn?
Java has a reputation for being a bit more difficult to learn than some other programming languages.
Hello World in Java
Here's an example of a simple Java program that prints Hello, World!
to the console:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, world!");
}
}
Output
Hello, World!
Is Java a open-source?
Java is partially open-source, with the Java Development Kit (JDK) being available under the GNU General Public License (GPL) version 2 with the Classpath Exception. However, other parts of the Java platform, including the Java Runtime Environment (JRE) and Java Virtual Machine (JVM), are not open-source.
Fun Fact

Did you Know?
Java was originally named Oak after a tree outside James Gosling's office, but the name was later changed to Java due to trademark issues. The name "Java" was chosen as a nod to the team's love of coffee.
Join our Community:
Author
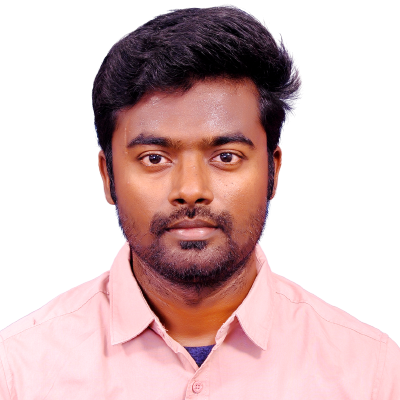
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Java Introduction) please comment here. I will help you immediately.